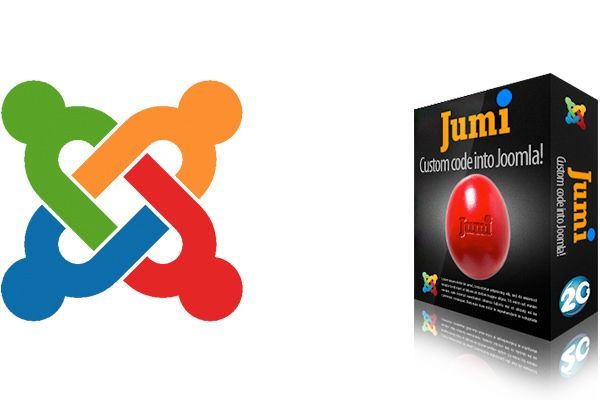
Jumi is a powerful extension that allows you to add custom PHP code in your site.
One way to use Jumi is to create custom PHP scripts that can be easily and safely used inside Joomla.
In this tutorial, I'm going to show you how to build Joomla database queries using the Jumi module.
Download and Install Jumi
Follow the guidelines from Step 1 in this previous post to download and install Jumi.
Build the database query
Create a file named custom.php with the code below:
<?php
// Connect to database
$db = JFactory::getDbo();
$query = $db->getQuery(true);
// Build the query
$query->select($db->quoteName(array('title', 'introtext')));
$query->from($db->quoteName('#__content'));
$query->where($db->quoteName('introtext') . ' LIKE '. $db->quote('%Joomla%'));
$query->order('ordering ASC');
$query->setLimit('1');
$db->setQuery($query);
$results = $db->loadObjectList();
// Print the result
foreach($results as $result){
echo '<h3>' . $result->title . '</h3>';
echo $result->introtext;
}
?>
Lets's break down that code to show you what it does.
First, we connect to the database safely, without needing to enter the database credentials:
$db = JFactory::getDbo();
$query = $db->getQuery(true);
Now we chose to query the title and introtext fields:
$query->select($db->quoteName(array('title', 'introtext')));
Next we have the name of a table. In this example, we have #__content table which is where the articles are stored.
$query->from($db->quoteName('#__content'));
Now we have the condition of the search. In this example we are looking in introtext for the word "Joomla".
$query->where($db->quoteName('introtext') . ' LIKE '. $db->quote('%Joomla%'));
Now we're going to choose the order of the search results; ascending or descending:
$query->order('ordering ASC');
Finally, we'll choose the maximum number of results:
$query->setLimit('1');
Create the Jumi module
- Upload the custom.php file to the root of your Joomla site.
- Go to Extensions > Module manager > New > Jumi.
- In the "Source of code" field, type the filename:

- Go to your public site to see the code in action. In this example, we pulled one article with the world "Joomla" in the title or introtext:
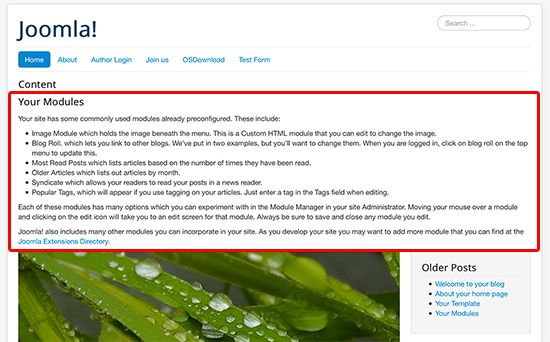